My last post was about getting a quick start in python. Now it would be much more interesting to apply the knowledge to a specific task. For this reason I decided to go through a really simple login system in python where a user can register and/or login using a username and a password.
Contents
Overview
Here I have a diagram showing the functionality our program will need. It can seem like a lot but it's really not that much.
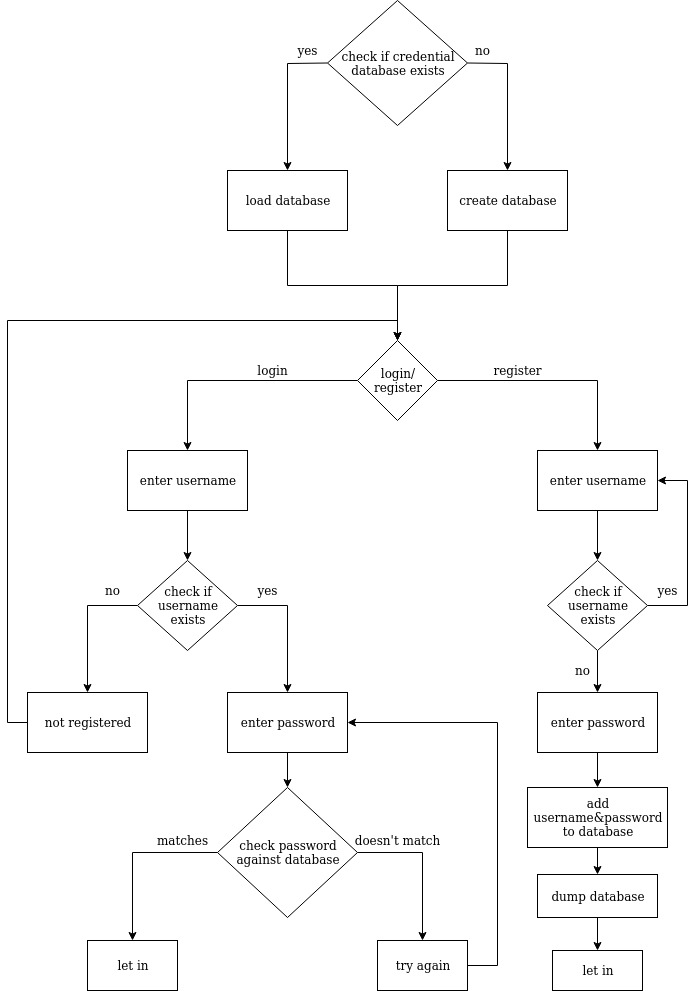
Let's go!
To get started let's create a file where we'll be writing the program (we need the filename to end with ".py"), then open the file with your favorite code editor, I'm currently using
Initializing everything we need
First we'll want to import (or load) all the libraries we'll need for the program. For this one we'll need json, os, and time which are all part of the standard python installation.
import json, os, time
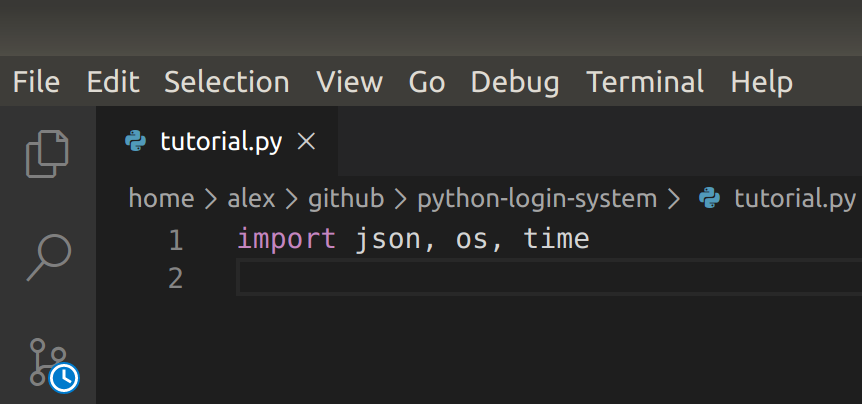
Let's set the variables. We'll make a dictionary called database where the credentials will be stored for the program runtime , and a allgood variable that we'll need to get out of loops later on.
database = {}
allgood = False
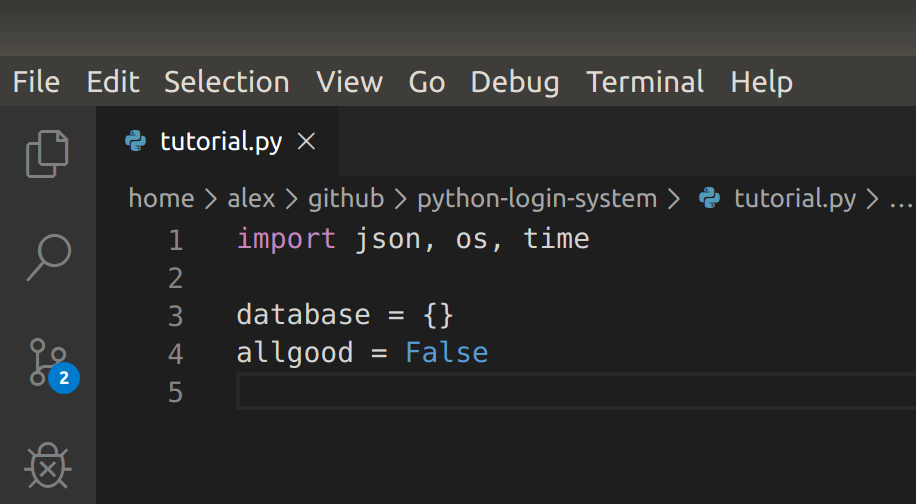
Check the database
Now the first thing in our diagram is to check whether the database already exists or not, then if it does, load it, if it doesn't, create it
if os.path.exists("database.json"):
with open("database.json", "r") as f:
database = json.load(f)
else:
with open("database.json", "w") as f:
json.dump(database, f)
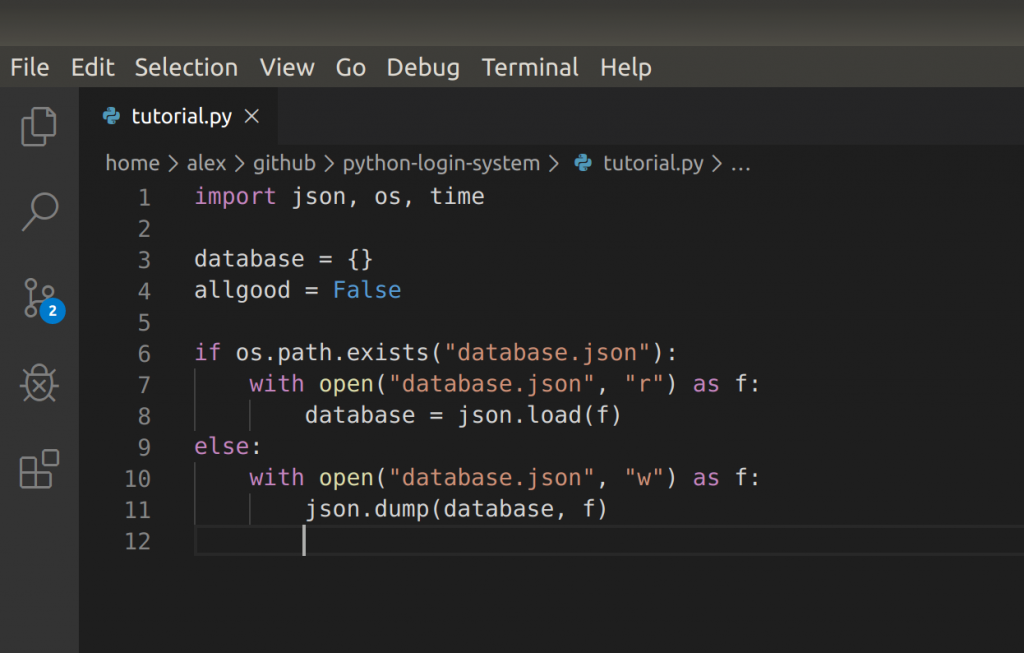
In our else statement we're dumping whatever we have (an empty dictionary) so that if the program is closed and opened again without anyone having registered then the database = json.load(f) doesn't fail.
Login or register?
Before we ask the user what they want to do, we need to implement a way for the user to come back to this menu we're going to make so let's make a loop and have everything happen inside it from now on.
while True:
And just for the sake of making it clean, let's put the while True inside a try statement so we can catch when the user exits the program and get rid of the traceback that usually comes with it.
try:
while True:
except KeyboardInterrupt:
print("losing")
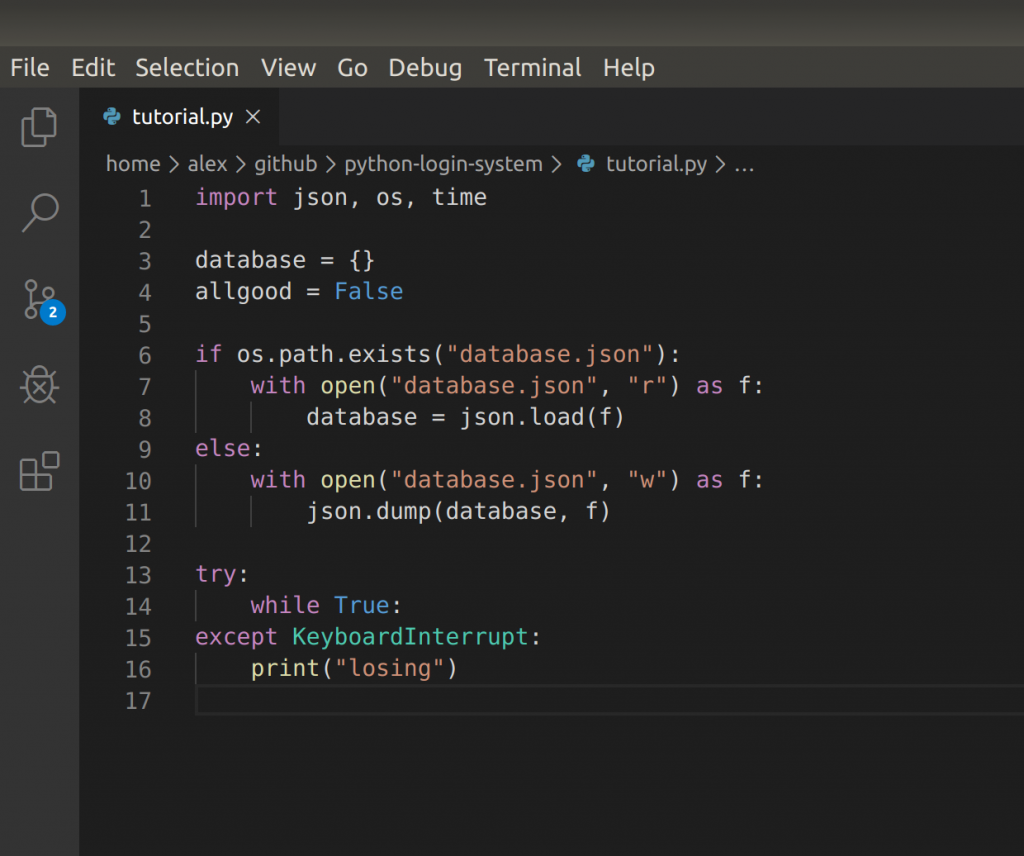
(I decided to just print losing in the terminal because it already displays ^C when you do Ctrl+C)
Also since we're getting to the user interface, let's clear the screen to make it look even more more clean and fancy.
os.system("clear")
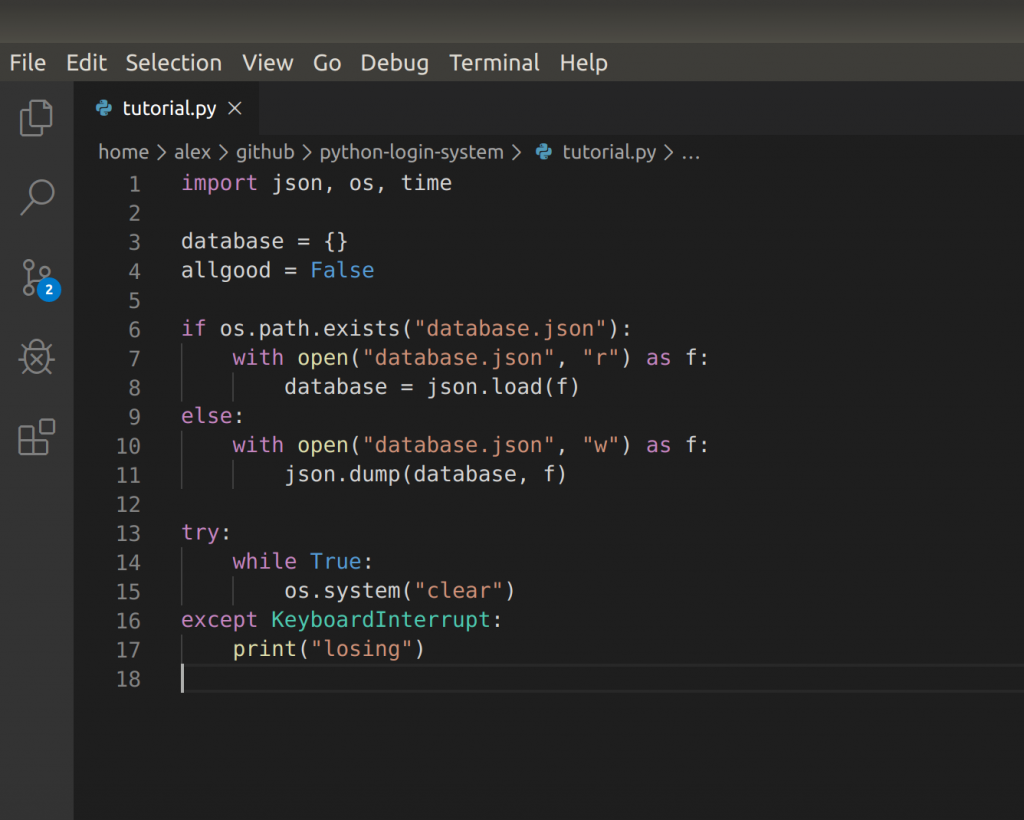
Now let's make a simple login/register menu
choice = input("0: login\n1: register\n\n")
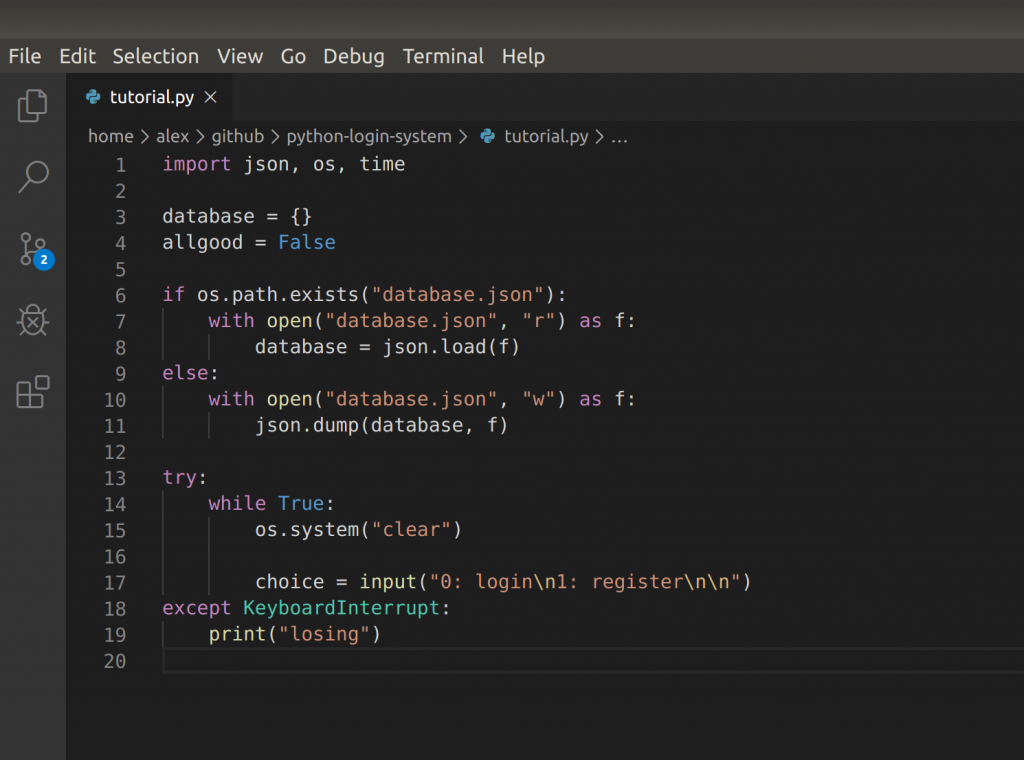
We specified 0 for login and 1 for register just to make it as little typing as possible for the user.
Now, let's interpret the user input.
if choice == "0":
# login
elif choice == "1":
# register
else:
print("Please type 0 or 1")
time.sleep(2)
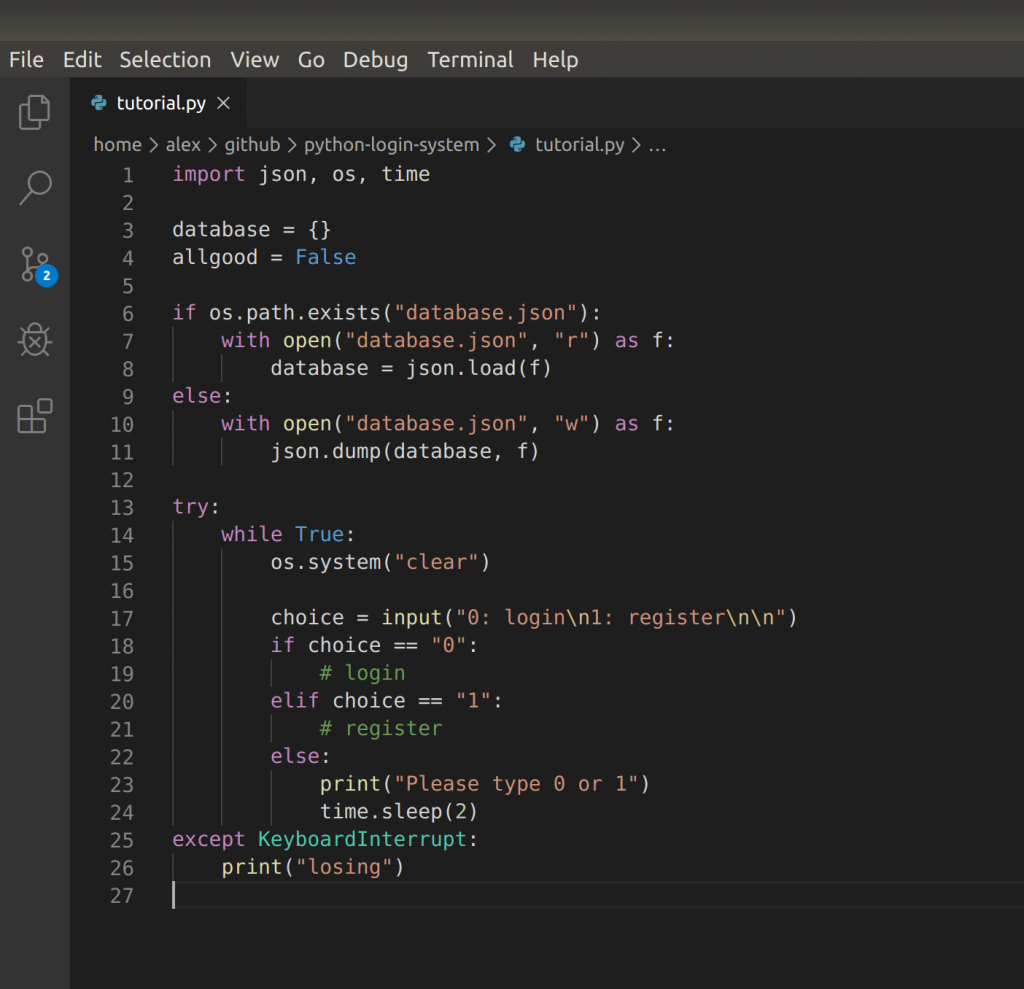
If the user types something other than what we expected it will simply bring them back to the menu while also telling them what they really need to do
Login
For the login we need to ask for the username then check if the username is in our database or not. Then if it's not in the database we tell the user about it.
os.system("clear")
username = input("username: ")
if not username in database:
print("username not found")
time.sleep(1.5)
else:
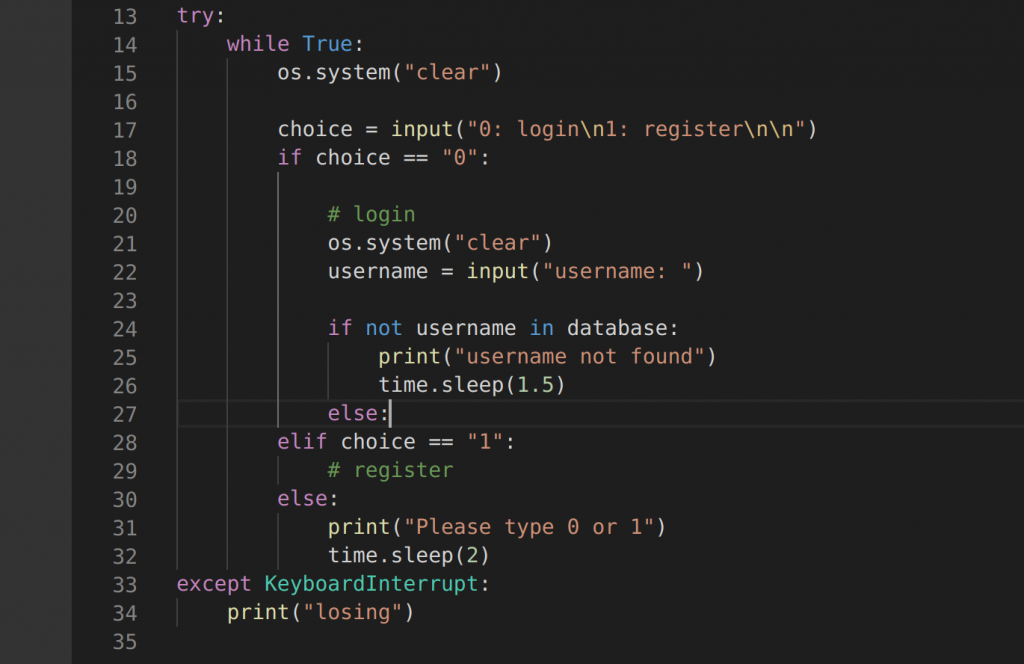
Now, if the username is in the database, we'll want to ask for the password, check it against the database and let's say we give them 3 tries to get the password right
for i in range(3):
password = input("password: ")
if password == database[username]:
allgood = True
break
elif i < 2:
print("incorrect password, try again")
if allgood:
break
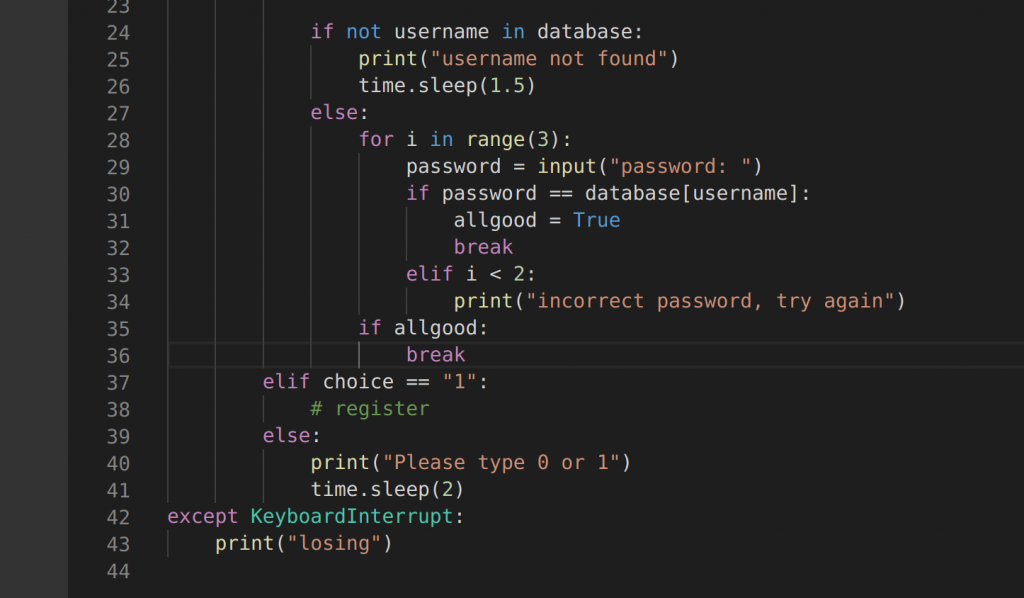
Notice since we're giving the three tries for the password we have a for loop that cycles 3 times, but we want to exit this loop and the main one if the user got the password right. So if they got it, we set the allgood variable to true then break. Then, right after the loop we check for the variable to be true, and if it is we exit the main loop as well.
Register
Our register part is going to be a little simpler because we don't need to check for the password to be correct, we simply take it and put it in our database.
So like in our login part, let's check the username against the database to make sure they're not registering under an existing username
os.system("clear")
while True:
username = input("username: ")
if not username in database:
else:
print("username already in use")
if allgood:
break
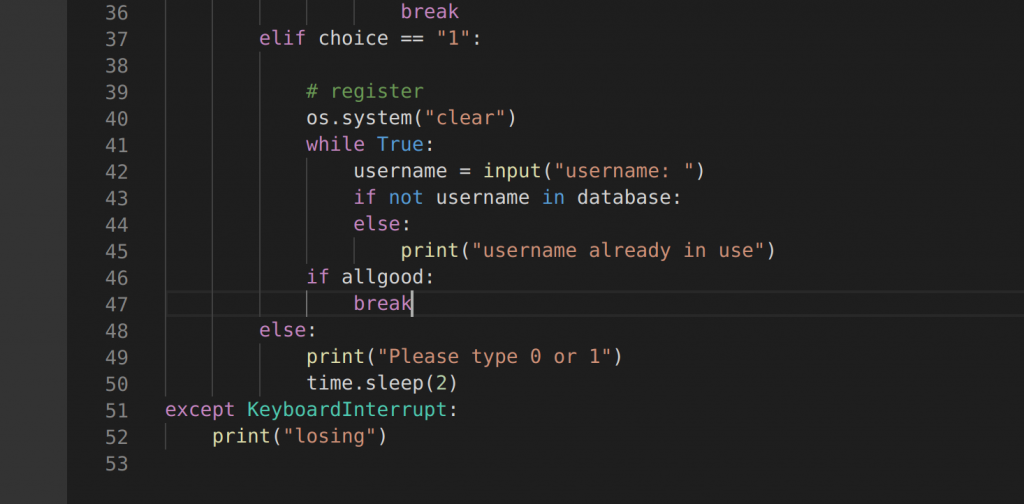
I also added the allgood check because again we're going to have a loop nested within a loop.
Now in the if statement where we make sure the username is not in the database, we get the password, store it all in our database, dump the database, and let the user in.
password = input("password: ")
database[username] = password
with open("database.json", "w") as f:
json.dump(database, f)
allgood = True
break
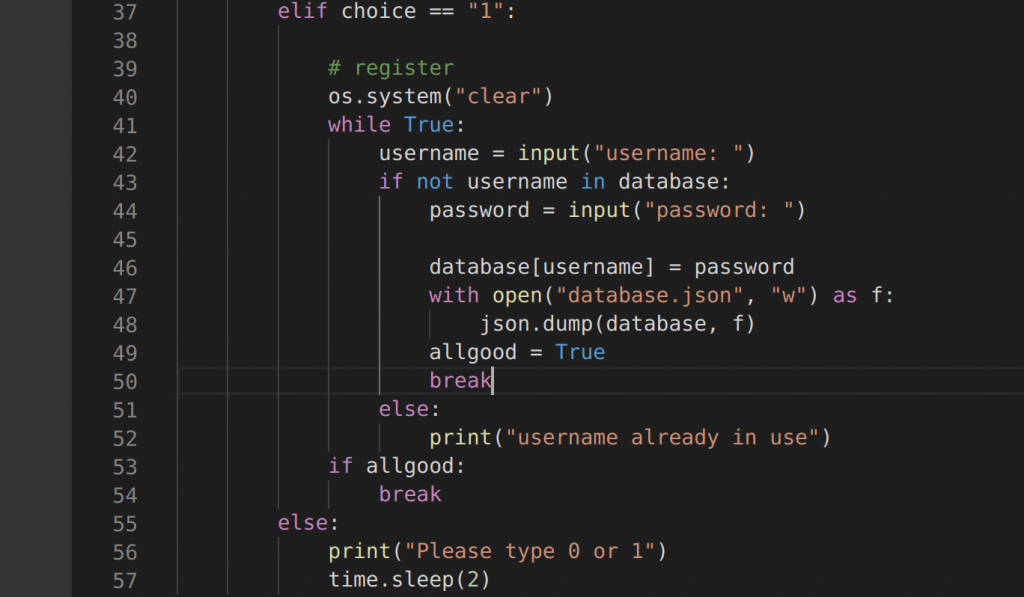
Done
We're done! Feel free to ask any questions you have in the comments.
The full code is on github: